Late Chunking: Improving RAG Performance with Context-Aware Embeddings
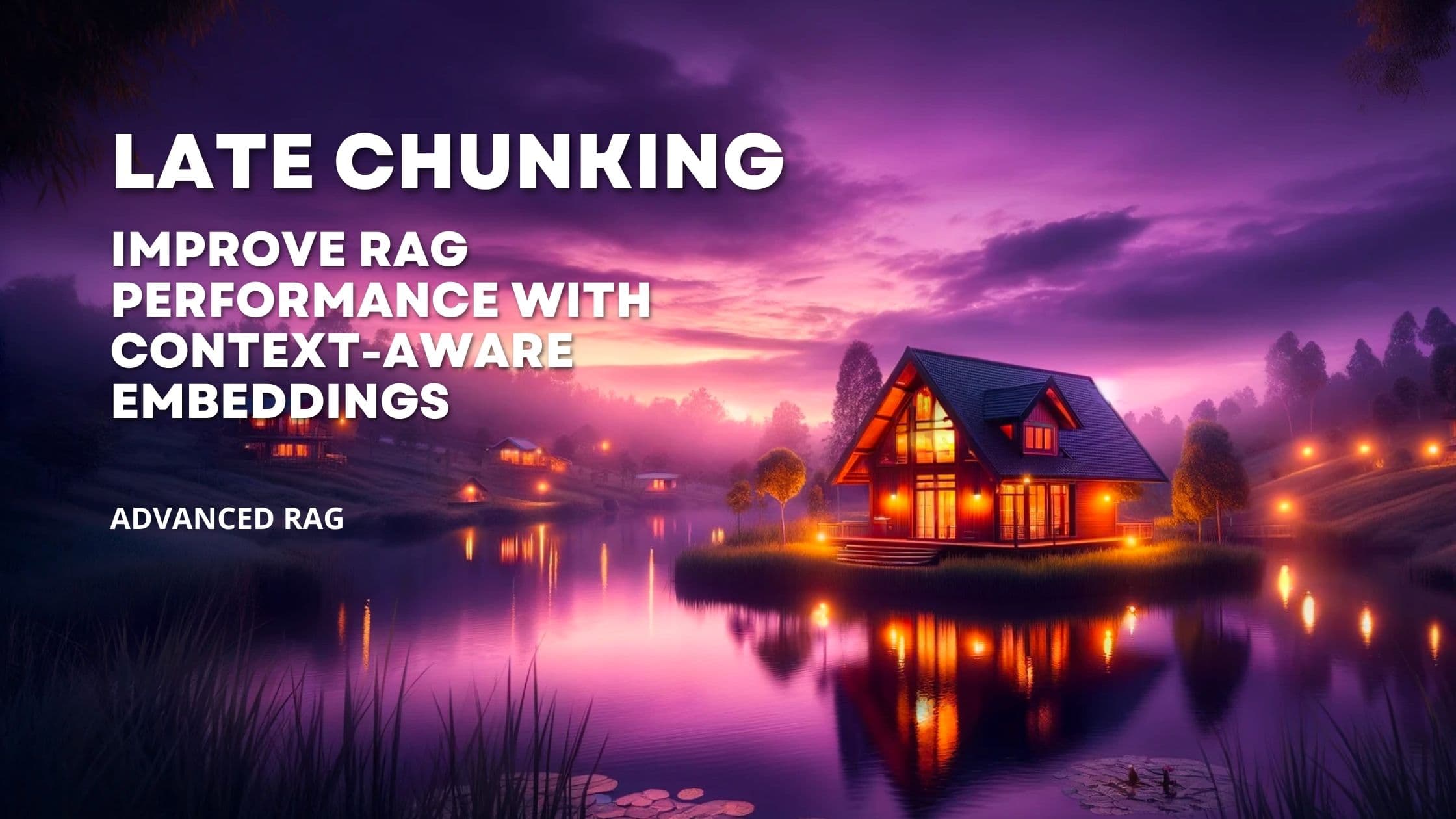
Retrieval Augmented Generation (RAG) has become a cornerstone of modern AI applications, but its efficiency often hinges on how well we handle document chunking.
When implementing RAG, developers must constantly balance between chunk sizes - too small, and we lose essential context; too large, and we compromise the embedding model's ability to capture precise semantic meaning. This isn't just a theoretical problem; it directly affects how accurately your system can retrieve relevant information for user queries.
Late chunking offers a practical approach to address this limitation. By processing documents through long-context embedding models before splitting them into chunks, we can maintain broader context while preserving semantic accuracy. In this guide, we'll examine the technical implementation of late chunking, compare its performance with traditional approaches, and provide concrete examples of how it improves retrieval quality.
For engineers and technical teams working with RAG systems, understanding this technique can lead to measurable improvements in retrieval accuracy.
NOTE: Late chunking was first introduced by the amazing people at jina ai. The code samples in this blog are also derived from their ground-work. And finally, we are going to use their outstanding jina-embeddings-v3 model for our examples. So shout-out to jina!
A quick introduction on Retrieval Augmented Generation
Retrieval Augmented Generation (RAG) is one of the most promising applications when it comes to LLMs. If you want a detailed introduction, please read our detailed post here.
RAG has two main phases:
-
The indexing phases
-
The retrieval phase
During indexing phase, the textual documents which are used as knowledge base for finding relevant information, are transformed to embeddings, which are high-dimensional vectors.
Embeddings are numeric representations of texts and roughly cover the semantic meaning of documents. The important thing is: Texts with similar semantic meaning have similar embeddings. For example, the text "My car" and "My automotive" have similar embeddings, whereas "My car" and "My cow" have rather different embeddings.
This fact can be used for advanced search algorithms, where users (or AI) can use natural language to search and find for semantically significant documents. That's basically the main element of RAG.
In the second phase, the retrieval phase, a user asks a question which should then be answered by an LLM. Using the embeddings created in phase 1 we can now search for documents which are semantically similar to the users question (and therefore are most likely to answer the users question).
Finally, we send the users question as well as the found documents to an LLM which then uses these docs to create a tailored answer.
A typical, simplified RAG pipeline is shown below:
Typical RAG pipeline
The problem with chunking in RAG applications
Now a rather big issue arises when we look at how (and why) we need to chunk our source documents.
Let's start with the why. Why do we need to chunk our documents in the first place? Simple, three reasons:
-
LLMs don't have unlimited context windows - meaning we need to limit the amount of data we send to the LLM. In an ideal world, we could simply send the users question alongside our whole knowledge base to the LLM and wait for the answer. Due to context limitations, this is not possible.
-
LLM context costs money. Even with LLM context windows getting bigger and bigger, each word we send to an LLM costs money. So we want to make sure that we somehow limit the costs our operation incurs.
-
LLMs have difficulties in finding the correct information in large quantities of data. If we have possibilities to pre-filter relevant information, the LLM answer will be better.
So, and now to the how: How does document chunking works? Well, there are various methods, but all of them boil down to the following: We need to divide the documents into chunk of length x words. We can do this by simply dividing by number of words or by dividing at semantically meaningful parts - like at the end of a paragraph or chapter.
However this introduces one significant issue: We will most certainly lose connected context, as we divide our document. Let's look at an example, let's say we have a document like:
"TensorFlow is one of the most popular open-source machine learning frameworks.
It was originally developed by researchers and engineers from Google Brain.
The framework provides a flexible ecosystem of tools, libraries, and community resources. These resources help developers build and deploy ML-powered applications efficiently.
The framework excels at numerical computation and large-scale machine learning. Its architecture allows for easy deployment of computation across various platforms."
Let's further assume we chunk the document after each paragraph (which in this case are mostly individual sentences, but bear with me, it will get clear that this problem persists independently from where you chunk).
Let's say we'd ask a question like: What framework was developed by Google Brain?
- we'd most probably find the second paragraph - however we
totally lost the context of which framework was created by Google Brain.
The word "It" is referring to "TensorFlow", however this
connection/context is lost when using paragraph-based chunking.
How does late chunking work?
Now that we know the problem, how can late chunking help to alleviate it?
As this is quite a complex topic, let's split it into two parts, a summary and a more detailed explanation:
Summary: Instead of first chunking our document into smaller parts and then creating embeddings for each of these parts, long chunking applies a model to the full document but then creates token-level embeddings (so, one embedding per token). These token-level embeddings are then combined to chunk-level embeddings (meaning, reduced to one embedding per chunk). As the initial embeddings were created on tokens of the whole text, each of them contains semantic meaning of the whole text, not just the token or chunks they represent. If we combine them later on to the chunked embeddings, each chunk not only knows about itself, but also surrounding junks.
(The chart below was kindly taken from jina ai)
Late chunking as per jina ai
Detailed description: The avid reader might not be satisfied with the superficial explanation above, so let's dive into more details. How does late chunking work in detail:
First, the text - the whole document if feasible - is tokenized (meaning it is divided in individual tokens):
Second, these tokens are passed through the model:
Now, the important part is, that embeddings are always the last hidden state of the model - so the last layer before the output. This means, we can simply access the embeddings of the tokens by accessing:
To make it more accessible, after passing the tokens through the model, we can access individual token embeddings by accessing the last_hidden_state of the model. This gives is something like below, if we use the example text provided above (Note, this representation is simplified, as it shows one embedding array per word, as in reality it would be one embedding array per token):
All we have to do, is to divide (speak, chunk) the array of embeddings. For example we can divide the array by looking at the token-text they represent. If, let's say' we chunk by sentence, we have to take the embeddings of all the tokens of the first sentence and create the vector average. This gives our final embedding for this chunk. Rinse and repeat for each and every sentence. The result is a list of chunk embeddings where each chunk embedding is derived from context-aware token embeddings, rather than processing each chunk in isolation.
The key difference from traditional chunking is that each token's embedding was created with awareness of the full context, so when "It" was embedded, the model already knew it referred to "Berlin" because it processed the whole text at once.
Why are these new chunks context-aware?
You might wonder, why these newly created chunks are more context-aware than with traditional chunking? The reason is the 'attention' - mechanism of the transformer architecture.
When the model processes "It", it doesn't just look at that token in isolation. Instead, through self-attention layers, each token can "attend to" (or "look at") all other tokens in the input text.
Here's a simplified explanation of how self-attention works:
-
For each token, the model calculates attention scores with every other token in the sequence. These scores represent how much attention should be paid to each other token when creating the final representation.
-
For example, when processing the word "It", the attention scores for the other words are:
-
The model learns these attention patterns during training. It learns that pronouns like "It" should pay strong attention to the nouns they refer to.
In code, this happens inside the transformer model when processing the full input:
The key difference to traditional chunking:
Traditional:
Late Chunking:
Interested in how to train your very own Large Language Model?
We prepared a well-researched guide for how to use the latest advancements in Open Source technology to fine-tune your own LLM. This has many advantages like:
- Cost control
- Data privacy
- Excellent performance - adjusted specifically for your intended use
Hans on: How to implement late chunking
Let's create an end to end example for our sample text above.
Now let's split the text into chunks of sentences. We need this for the traditional method. We also need this, to calculate the positions of the tokens after which we want to chunk in our new method. (Remember, we need to chunk our array of embeddings after we created the embeddings for the whole text. We can do this, by remembering the start and end of our sentences).
Lust but not least we can create our late chunking implementation:
That's it, now we can use our methods to create embeddings as follows:
To compare these two methods, let's create a small 'benchmark' where we output the similarity between the word "TensorFlow" with all the chunks:
These are the results in our case:
If you look at the similarity scores (the higher, the better), the results are mind-blowing. While the similarity score drops a bit in the initial chunk, it is enormously better in all the other chunks.
It's to be expected, that the score drops a bit for the first chunk, as the 'traditional' method is already very good for finding chunks where the search term directly represents the chunk.
For all the other chunks, it's clear, that they are not 'semantically' connected to the first chunk, which - depending on your use case - can and will boost your RAG search performance.